Recent Posts
- How-to: Unlock Git Metadata Correctly With In AWS CodeBuild
- Using AWS Secrets Manager In AWS CodeBuild: A Beginner’s Guide
- Simplified Excellence: Your Ultimate Guide to Hosting a Static Website
- Domain Registration Made Easy: A Comprehensive AWS Guide
- Simplifying NVM Integration In AWS CodeBuild For Node.js Projects
Archives
Categories
How-to: Unlock Git Metadata Correctly With In AWS CodeBuild
Imagine you’re in the vast world of AWS Cloud Services, a place filled with tools to make your cloud journey seamless. Picture a scenario where you have CodeCommit as your source, a CodeBuild instance for building, and CodeDeploy for deploying your application. It’s like assembling a powerful team under one roof, with CodePipeline orchestrating the entire process.
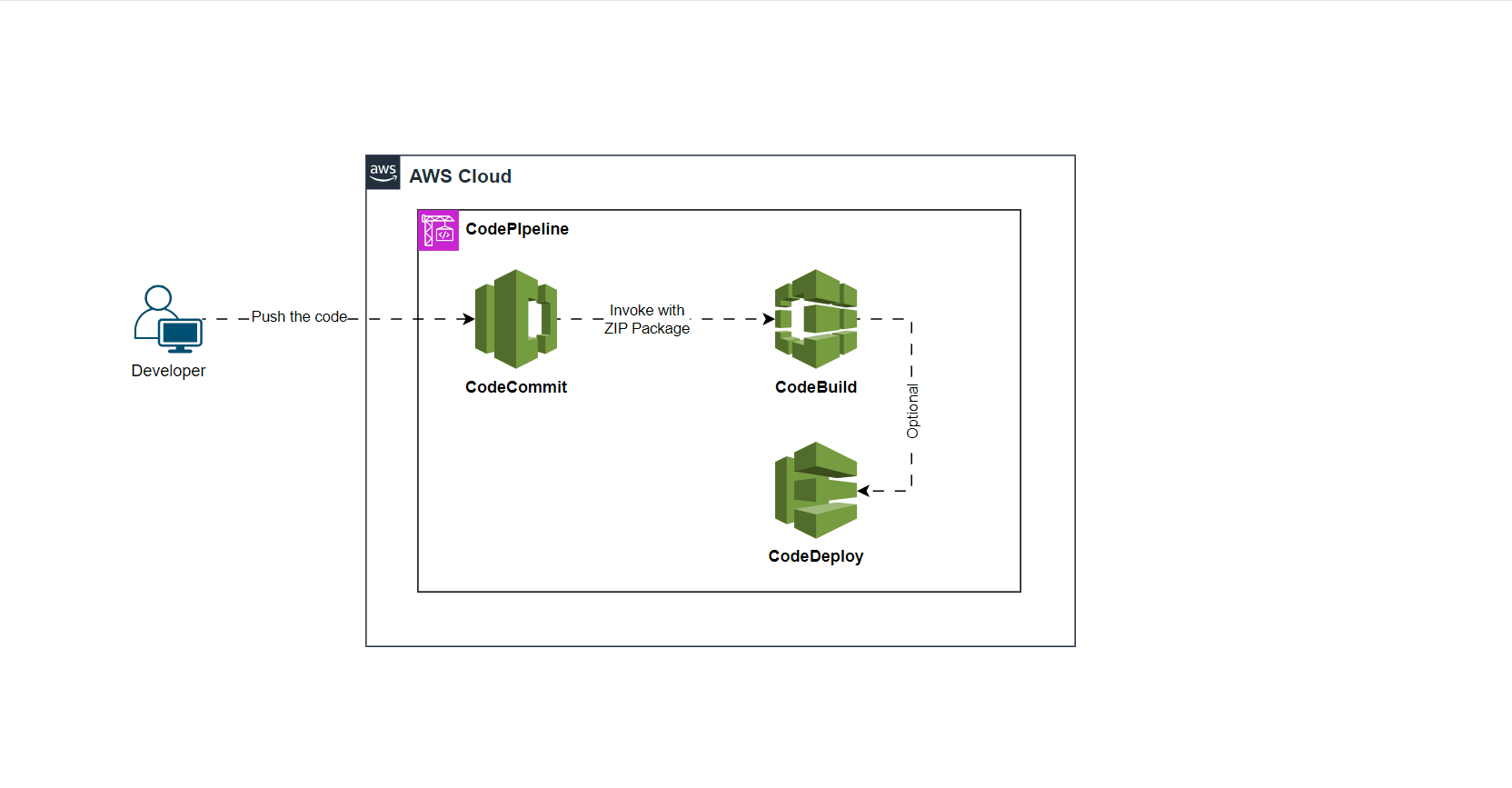
Everything is running smoothly until the day you decide to harness the power of Git metadata—details about your code commits. You want to automate linking commit information to specific development stories in your ticket management board, like JIRA. You add a simple Git command to your build process, and suddenly, an error appears.
Now, the quest begins: How do you overcome this challenge and effectively use Git metadata without turning your architecture upside down or slowing down your builds? If you’ve landed here seeking answers, you’re in the right place.
But first, let’s understand why CodeBuild doesn’t play nice with Git commands by default. When setting up a CodePipeline integrating CodeCommit and CodeBuild, AWS recommends using “CODE_ZIP.” Why? ZIP archives are like compressed magic—making data transfer efficient and simplifying the process with a single compressed file. ZIP is the go-to for packaging and distributing code for deployment.
Now, let’s dive into the solution step by step:
Step 1: Update the CodePipeline
Navigate to the AWS CodePipeline service within the AWS Management Console. Kickstart the process of updating your pipeline because, in the ever-evolving cloud, change is the only certainty. If you’re seeking expert guidance on creating a pipeline, check out this blog link—it’s your ticket to mastering the art.
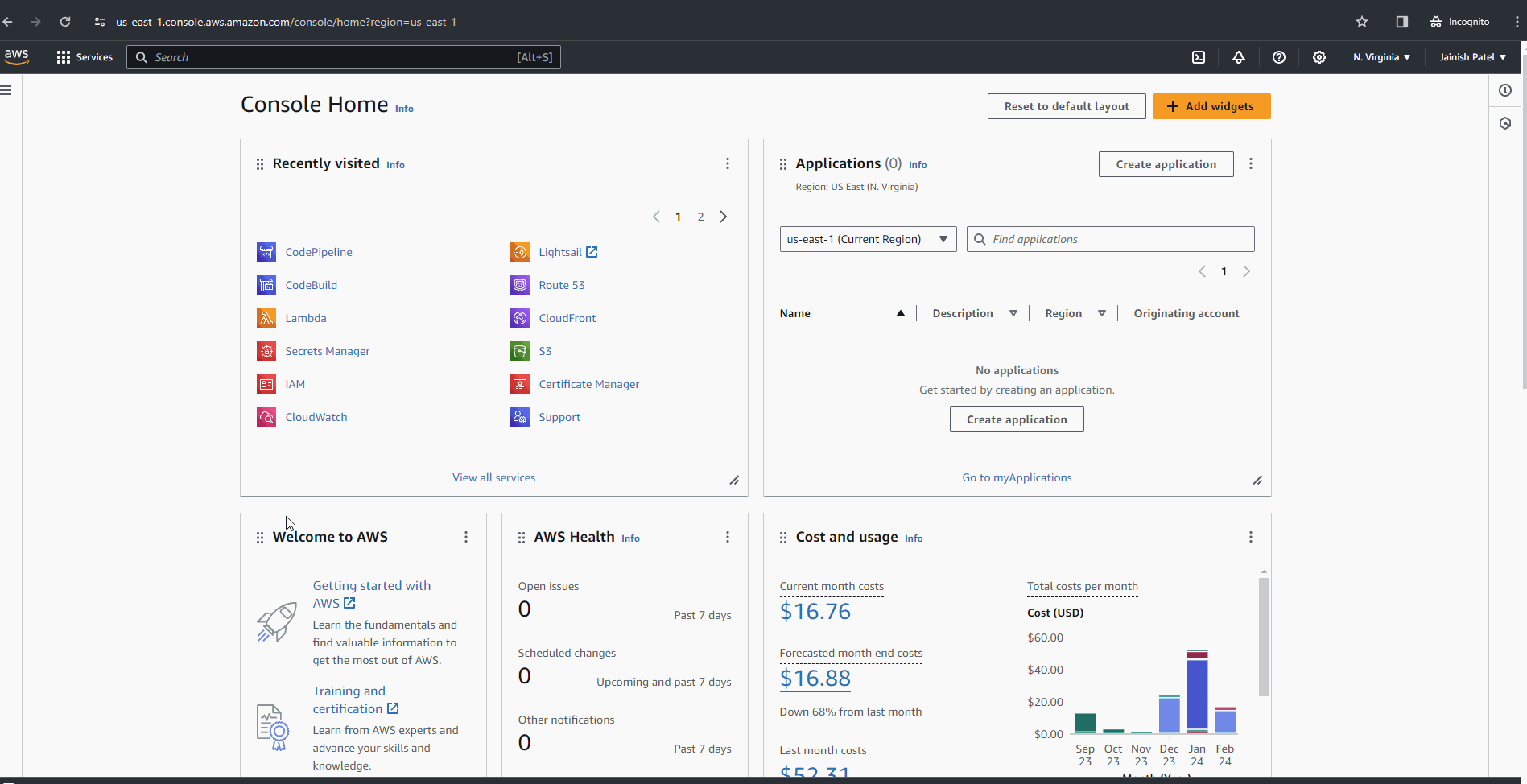
Step 2: Boost CodeBuild IAM Permissions
Navigate to AWS CodeBuild, locate your build project and IAM role. Elevate permissions by adding the provided IAM magic and replace the <AWS::Region>, <AWS::AccountID>, and <Codecommit Repository Name>
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Resource": [
"arn:aws:codecommit:<AWS::Region>:<AWS::AccountID>:<Codecommit Repository Name>"
],
"Action": [
"codecommit:GitPull",
"codecommit:GetRepository"
]
}
]
}
Step 3: Craft Your CodeBuild Buildspec
version: 0.2
phases:
pre_build:
commands:
- git log | head -2
- commit_message=$(git log -1 --pretty=%B)
- echo "Commit message is $commit_message"
- git status
Picture this: You’re on a thrilling cloud adventure, building pipelines and deployment packages that are like intricate puzzles. But, oh no! The complexity is through the roof, and you dread making changes to your trusted BuildSpec file. You fear breaking the code and spending extra hours fixing what was once a masterpiece.
But fear not, brave coder! A solution awaits, one that won’t disrupt your coding zen. Let’s unravel this challenge together.
Step 1: Boost CodeBuild IAM Permissions
Navigate back to AWS CodeBuild, find your build project, and update the IAM role with the IAM sorcery provided.
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Resource": "*",
# You can replace the * with your specific Pipeline ARN.
"Action": [
"codepipeline:ListActionExecutions"
],
}
]
}
Step 2: Craft Your CodeBuild Buildspec
version: 0.2
phases:
pre_build:
commands:
- codepipeline_name=${CODEBUILD_INITIATOR#codepipeline/}
- echo "CodePipeline name is $codepipeline_name"
- commit_message=$(aws codepipeline list-action-executions --pipeline-name $codepipeline_name | jq -r --arg commitId "$CODEBUILD_RESOLVED_SOURCE_VERSION" '.actionExecutionDetails[] | select(.output.outputVariables.CommitId == $commitId) | .output.outputVariables.CommitMessage')
- echo "Commit Message is $commit_message"
Pay attention! Once you run the command above, it spills out a payload—a digital treasure trove. To extract the gem you seek, think of the “jq” command as your trusty filter tool. In the command snippet I shared, witness the magic of “jq” unraveling the payload, revealing the coveted latest commit message. Behold the power of code in action!
{
"actionExecutionDetails": [
{
"pipelineExecutionId": "EXAMPLE0-adfc-488e-bf4c-1111111720d3",
"actionExecutionId": "EXAMPLE4-2ee8-4853-bd6a-111111158148",
"pipelineVersion": 12,
"stageName": "Deploy",
"actionName": "Deploy",
"startTime": 1598572628.6,
"lastUpdateTime": 1598572661.255,
"status": "Succeeded",
"input": {
"actionTypeId": {
"category": "Deploy",
"owner": "AWS",
"provider": "CodeDeploy",
"version": "1"
},
"configuration": {
"ApplicationName": "my-application",
"DeploymentGroupName": "my-deployment-group"
},
"resolvedConfiguration": {
"ApplicationName": "my-application",
"DeploymentGroupName": "my-deployment-group"
},
"region": "us-east-1",
"inputArtifacts": [
{
"name": "SourceArtifact",
"s3location": {
"bucket": "artifact-bucket",
"key": "myPipeline/SourceArti/key"
}
}
],
"namespace": "DeployVariables"
},
"output": {
"outputArtifacts": [],
"executionResult": {
"externalExecutionId": "d-EXAMPLEE5",
"externalExecutionSummary": "Deployment Succeeded",
"externalExecutionUrl": "https://myaddress.com"
},
"outputVariables": {}
}
},
{
"pipelineExecutionId": "EXAMPLE0-adfc-488e-bf4c-1111111720d3",
"actionExecutionId": "EXAMPLE5-abb4-4192-9031-11111113a7b0",
"pipelineVersion": 12,
"stageName": "Source",
"actionName": "Source",
"startTime": 1598572624.387,
"lastUpdateTime": 1598572628.16,
"status": "Succeeded",
"input": {
"actionTypeId": {
"category": "Source",
"owner": "AWS",
"provider": "CodeCommit",
"version": "1"
},
"configuration": {
"BranchName": "production",
"PollForSourceChanges": "false",
"RepositoryName": "my-repo"
},
"resolvedConfiguration": {
"BranchName": "production",
"PollForSourceChanges": "false",
"RepositoryName": "my-repo"
},
"region": "us-east-1",
"inputArtifacts": [],
"namespace": "SourceVariables"
},
"output": {
"outputArtifacts": [
{
"name": "SourceArtifact",
"s3location": {
"bucket": "my-bucket",
"key": "myPipeline/SourceArti/key"
}
}
],
"executionResult": {
"externalExecutionId": "1111111ad99dcd35914c00b7fbea13995EXAMPLE",
"externalExecutionSummary": "Edited template.yml",
"externalExecutionUrl": "https://myaddress.com"
},
"outputVariables": {
"AuthorDate": "2020-05-08T17:45:43Z",
"BranchName": "production",
"CommitId": "EXAMPLEad99dcd35914c00b7fbea139951111111",
"CommitMessage": "Edited template.yml",
"CommitterDate": "2020-05-08T17:45:43Z",
"RepositoryName": "my-repo"
}
}
},
. . . .
Source: AWS Documentation
Congratulations, you’ve unleashed the power of Git in AWS CodeBuild! Your cloud journey just got a whole lot more exciting.